Solving the Doctype Filter Insufficiency in Oracle WebCenter Sites
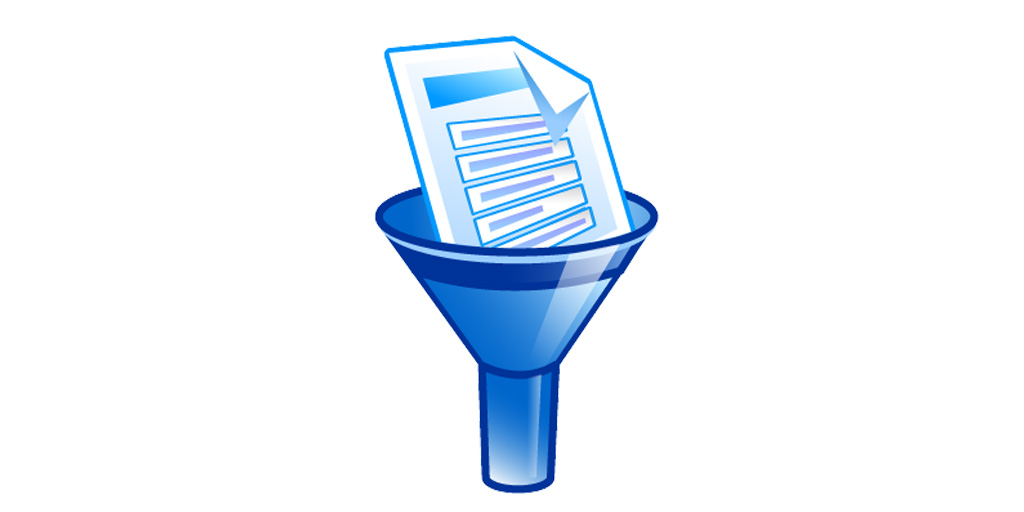
I bet that you have once created an asset, with a doctype filter, uploaded a file as an attribute, and noticed that the filetype and mimetype were not getting pulled. You checked the file once again to see that it had an extension and was actually not corrupted either. Everything seemed ok. You edited the asset. Re-saved it. Shook your head. Sweated a bit. Checked the asset definition to make sure that the doctype filter was still associated with the definition. Checked the doctype filter's attribute names. Well, you never know... a typo here and there... Nope. Nothing. You just don't know what to do.
Well here's a small tip that will save you time. The FileType java class is where the magic happens. The only problem is that the magic is not magical enough to handle as many file types.
Looking through the code, we can see these lines constructing a hashmap of mime types:
Map m = new HashMap(); m.put("pdf", "application/pdf"); m.put("latex", "application/x-latex"); m.put("ltx", "application/x-latex"); m.put("tcl", "application/x-tcl"); m.put("tex", "application/x-tex"); m.put("man", "application/x-troff-man"); ..... file2mt = Collections.unmodifiableMap(m);
And another set of lines mapping the filename's extension to a potential file type:
int i = s1.length(); int j = s1.lastIndexOf(".") + 1; String s2 = s1.substring(j, i); String s3; if (s2.equalsIgnoreCase("DOC")) s3 = "Word Document"; else if (s2.equalsIgnoreCase("xls")) s3 = "Excel Spreadsheet"; else if (s2.equalsIgnoreCase("pdf")) s3 = "PDF"; else if (s2.equalsIgnoreCase("HTML")) s3 = "HTML"; .... return s3
This will work, in a very limited way. As long as your uploaded files in WCS belong to the out-of-the-box list of extensions handled in the FileType.java file, you shall be ok. However, for every new extension required, you would have to explode the cs.jar, find the Filetype.java file and add a new hash value/pair. Compile. Rebundle. Redeploy. There has to be a better way to handle new additions and old changes. When you start thinking about it a bit more, your mind wanders towards the MimeType database table. Why not use this table instead? It does contain extensions and file information. Shouldn't these mime and file types be retrieved from this table? We could use the mimetype column for mime types and the extension column for file types.
Thus our solution shapes up. What if we:
1. Create a row in the MimeType table for each extension file
2. The mimetype column will contain the mime type of the said extension
3. The keyword column will contain the file type of the said extension
Let's give it a try.
First let's add a couple of extension rows:
Now let's turn to modifying the FileType.java code.
Instead of:
public FileType(String inval) { setFilename(inval); setFiletype(getFileType(inval)); setFileext(inval); setMimetype(getMime(getExt(inval))); }
First we are generating an ics session that will allow us to query the MimeType table at will while setting up the mimetype (setMimetype) and filetype (setFiletype) variables:
public FileType(String inval){ String ext = getExt(inval); getSession(ics); setFilename(inval); setFiletype(getTableData("keyword",ext)); setFileext(inval); setMimetype(getTableData("mimetype",ext)); }
In String getTableData(String column, String extension), we are querying for the column's value specified based on the extension provided:
private String getTableData(String column, String extension){ String columnrow = null; String extensionLower = extension.toLowerCase(); Map data = new HashMap(); try { java.util.ArrayList<String> mimetypeList = new java.util.ArrayList<String>(); mimetypeList.add("MimeType"); String sqlStr="SELECT extension, " + column + " FROM MimeType where extension='" + extensionLower + "'"; PreparedStmt colStatement = new PreparedStmt(sqlStr, mimetypeList); StatementParam noParam = colStatement.newParam(); IList resultsList = ics.SQL(colStatement, noParam, true); if (resultsList!=null && resultsList.hasData()){ String emptycolumnrow = "Unknown "; emptycolumnrow = (column.equals("keyword")) ? emptycolumnrow + " Filetype" : emptycolumnrow + column; resultsList.moveTo(1); columnrow = resultsList.getValue(column); if(columnrow.isEmpty()) columnrow = emptycolumnrow; data.put(extensionLower, columnrow); } } catch(Exception e) { log.error("get " + column + ": Cannot retrieve data information from MimeType table"); e.getStackTrace(); } String mapped = (String) data.get(extensionLower); return mapped; }
All in all, future additions only require adding new rows to the MimeType database table. This can be done via the support tools.
There....You will no longer have to add new mime/file type information to the java file. Happy asset creations!
- Log in to post comments